StringBuilder is a tool for building and changing text. It’s a flexible container for words where you can easily add, remove, or rearrange letters. It’s faster than a similar tool called StringBuffer, especially when working alone. You can use it to combine different types of data into text, and it’s perfect for creating long or complex strings without slowing down your program. The following article will elaborate on the StringBuilder Java methods in detail. In this Java StringBuilder tutorial, you will learn how to utilize all the StringBuilder methods with practical examples. This enables you to efficiently manage and manipulate strings in your Java programs.
Understanding Java StringBuilder Class
You can use either StringBuilder Java methods or a StringBuffer to do this. Both let you add, remove, or change blocks as you go. However, there’s a key difference: speed. StringBuilder is like a speedy builder working alone, while StringBuffer is like a slower builder who double-checks everything to ensure no one else messes up the sentence.
So, if you’re the only one building the sentence (or working with your code in a single thread), use StringBuilder for a faster build. But if multiple threads) work on the sentence together, and use StringBuffer to avoid mistakes.
Syntax:
public final class StringBuilder
extends Object
implements Serializable, CharSequence
How to Create Java StringBuilder Class?
There are different ways to create StringBuilder class in Java:
- StringBuilder(): You can start with empty StringBuilder java methods. However, this is the default size, which can hold up to 16 characters.
- StringBuilder(int capacity): You can choose the size of your canvas upfront. If you know you’ll be writing a long text, you can make the StringBuilder method in Java ample to avoid running out of space.
- StringBuilder(CharSequence seq): You can start with a picture (or text) and copy it onto your StringBuilder java. However, it is worthwhile if you want to modify existing text.
- StringBuilder(String str): Creates a string builder that is initialized with the contents of the specified string.
So, you have options, to begin with an empty java StringBuilder of your preferred size or one that already has something on it.
Types of StringBuilder Java Methods
StringBuilder provides a variety of methods to manipulate text. Let’s elaborate on them in the upcoming section.
- StringBuilder append(X x): This method adds the string representation of the argument of type X to the sequence.
- StringBuilder appendCodePoint(int codePoint): This approach adds the string representation of the codePoint argument to this sequence.
- Int capacity(): It returns the current capacity.
- Char charAt(int index): It efficiently retrieves the character value in this sequence at the specified index.
- IntStream chars(): This method unequivocally returns a stream of integer values zero-extending the character values from this sequence.
- Int codePointAt(int index): This StringBuilder Java method provides the character (Unicode code point) at the given index.
- Int codePointBefore(int index): It efficiently retrieves the Unicode code point of the character preceding the specified index.
- Int codePointCount(int beginIndex, int endIndex): This method returns the number of Unicode code points in the specified text range of this sequence.
- IntStream codePoints(): This procedure returns a sequence of code point values from this string and maintains Java StringBuilder performance.
- StringBuilder delete(int start, int end): Highly effective StringBuilder Java method efficiently eliminates characters within a specified substring of this sequence.
- StringBuilder deleteCharAt(int index): It definitively removes the character at the specified position in this sequence.
- Void ensureCapacity(int minimumCapacity): It guarantees that the capacity is at least as large as the specified minimum.
- Void getChars(int srcBegin, int srcEnd, char[] dst, int dstBegin): In this efficient method, characters are seamlessly transferred from this sequence into the designated character array, dst.
- Int indexOf(): It returns the index of the first occurrence of the specified substring within the string.
- StringBuilder insert(int offset, boolean b): It forcefully inserts the string representation of the boolean alternate argument into this sequence.
- StringBuilder insert(): This StringBuilder class in Java elegantly incorporates the string representation of the character argument into this sequence.
- Int lastIndexOf(): It returns the index of the last occurrence of the specified substring within this string.
- Int length(): This function returns the length of the string, the character count.
StringBuilder Java Methods Examples
The following section will elaborate on the Java StringBuilder examples.
Example 1:
public class StringBuilderExample {
public static void main(String[] args) {
StringBuilder sb = new StringBuilder();
sb.append(“Hello”);
sb.append(” “);
sb.append(“world!”);
System.out.println(sb.toString()); // Output: “Hello world!”
sb.insert(6, “beautiful “);
System.out.println(sb.toString()); // Output: “Hello beautiful world!”
sb.reverse();
System.out.println(sb.toString()); // Output: “!dlrow lufituaeb olleH”
}
}
Example 2:
Input :
StringBuilder = I love my Country
char a = A
Output: I love my Country A
Example 3:
Input:
string_buffer = “We are Indians”
boolean a = true
Output: We are Indians true
Also Read: Checkout 20 Advantages and Disadvantages of Technology in Education
Wrapping Up Thoughts!
StringBuilder is a powerful tool in Java for efficiently manipulating strings. Unlike immutable Strings, StringBuilder allows you to modify its contents directly. As a result, it makes it ideal for building and modifying text dynamically. With a rich set of methods for appending, inserting, deleting, and modifying characters, StringBuilder Java methods offer flexibility and performance advantages over traditional string concatenation. While it excels in single-threaded environments, it’s essential to use StringBuffer for thread-safe operations.
Frequently Asked Questions (FAQs)
Q. Why is StringBuilder mutable in Java?
Ans. StringBuilder in Java is a changeable piece of text. Unlike regular strings, which can’t be altered once created, StringBuilder lets you add, remove, or change letters and words as often as you need.
Q. Does StringBuilder have a Contains method?
Ans. No! StringBuilder does not have a contains approach.
Q. What is the difference between string methods and StringBuilder methods?
Ans. StringBuilder is faster than regular String for building and changing words. When you change regular text, it’s like creating a whole new piece of text every time, which is slow. But StringBuilder is like editing a single piece of text over and over. However, it makes it much quicker, especially when you’re working with lots of words or changing text frequently.
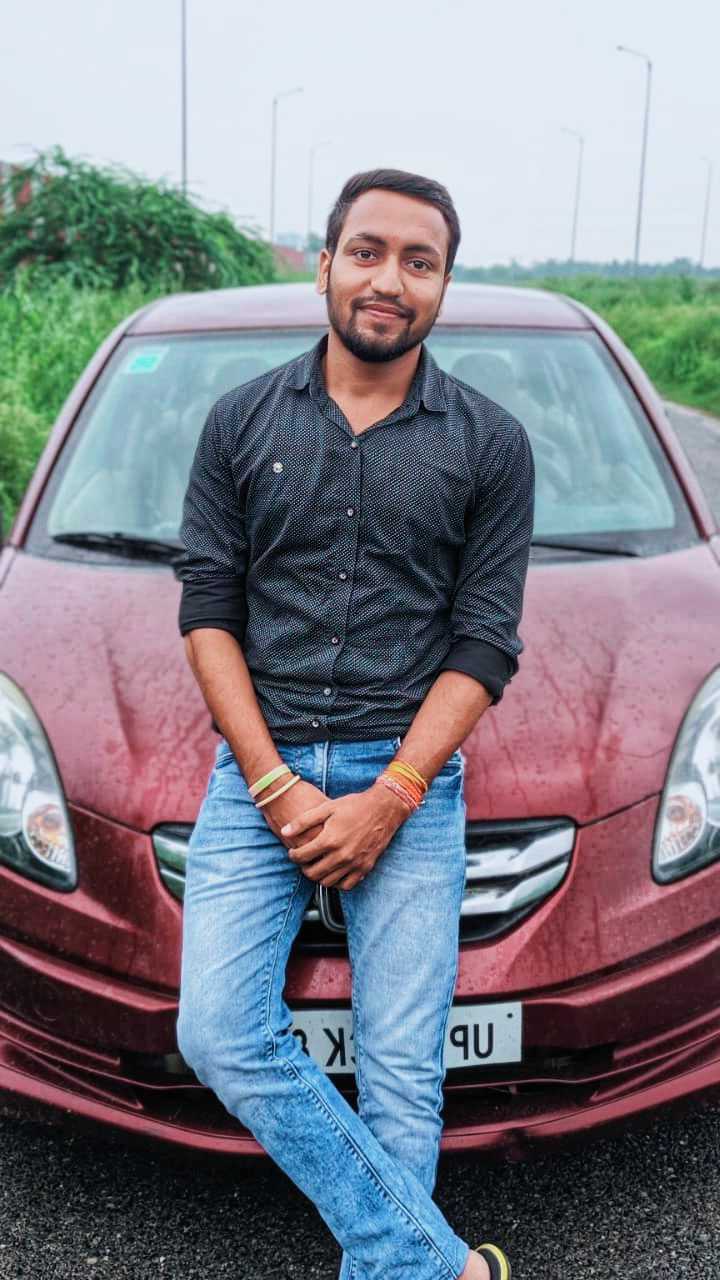
Ankit Roy is a professional Technical Content Developer, Freelancer, and blogger. He holds a Masters’s degree in Computer Science and has worked as a Digital Marketing Strategist. With a deep understanding of technology trends like AI, ML, Big Data, Neural Networks, Network Infrastructure, etc., Ankit is able to communicate complex technical concepts in a clear and concise manner. He is a regular contributor to several websites and has authored numerous technical guides and instructional materials. In his free time, Ankit enjoys tinkering with new technologies and staying up-to-date with the latest developments in the field.